Chart.js is an open-source JavaScript library that renders in HTML5 canvas. It is used to create HTML-based charts. This library was launched in 2013 by Nick Downie. Currently, it is the most popular charting library in 2022. In this post, I will talk about Angular Chart.js integration.
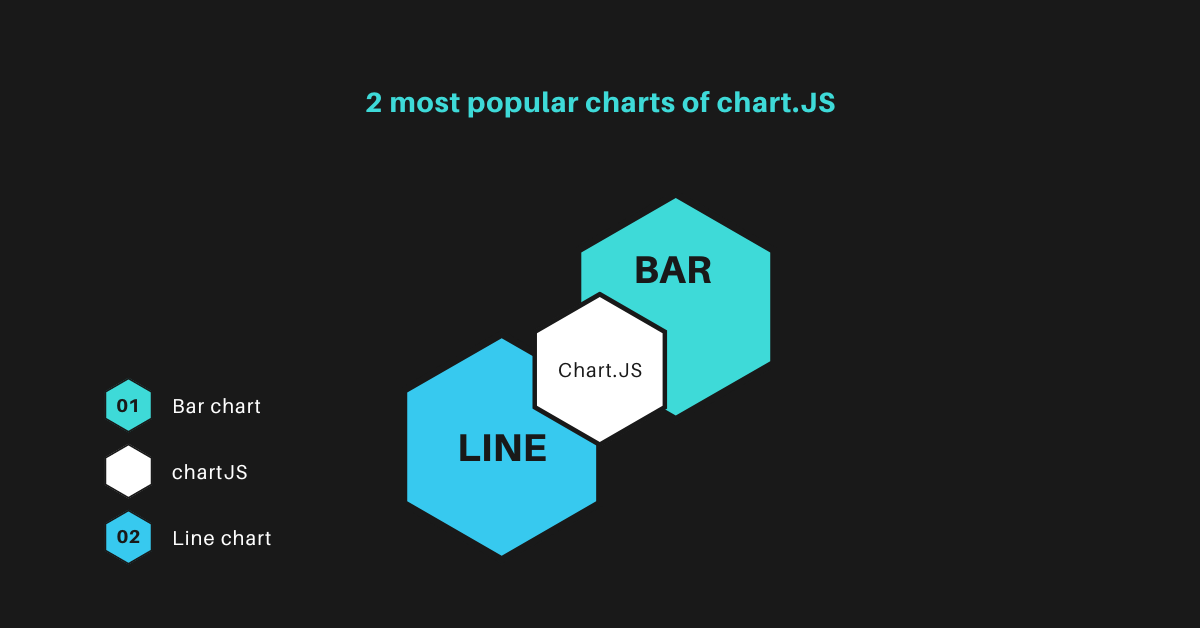
Preparing your Angular app
Do you already have an app? If not, you can easily create it by the command:ng new angular-chartjs
Now that you’ve created your angular application, navigate to the project folder and start the local development server using the command: cd angular-chartjs && ng serve -o
Installing Chart.js
Installation of Chart.js is very simple. Navigate to your project directory and run: npm install chart.js
Chart.js is now added to your Angular app!
Generating new components
Now we need to create two angular components:
- one for the Bar chart
- the other one for the Line chart
We will do this by running two commands:
ng generate component bar-chart
ng generate component line-chart
Creating a Bar Chart with Angular Chart.js
A bar graph or chart displays categorical data using rectangular bars with heights or lengths proportional to the values they represent. The bars can be plotted either vertically or horizontally. A column chart is another name for a vertical bar graph.
Adding a Bar chart
Paste this HTML code in bar chart HTML component:
<div class="chart-container">
<canvas id="MyChart" >{{ chart }}</canvas>
</div>
Now add chart js library in bar chart Typescript component:
import Chart from 'chart.js/auto';
//or
import Chart from 'chart.js';
This variable will hold the information of our graphs:
public chart: any;
Now we will create a method for the bar chart. This will include the data and labels for our bar chart. Copy the following code and paste it in the bar-chart.component.ts
file below the ngOnInit()
function:
createChart(){
this.chart = new Chart("MyChart", {
type: 'bar', //this denotes the type of chart
data: {// values on X-Axis
labels: ['2022-05-10', '2022-05-11', '2022-05-12','2022-05-13', '2022-05-14', '2022-05-15', '2022-05-16','2022-05-17'],
datasets: [
{
label: "Sales",
data: ['467','576', '572', '79', '92', '574', '573', '576'],
backgroundColor: 'green'
},
{
label: "Profit",
data: ['542', '542', '536', '327', '17','0.00','538', '541'],
backgroundColor: 'blue'
}
]
},
options: {
aspectRatio:2.5
}
});
}
We are providing the type of chart, the labels, and the data in this code.
We want our createChart()
function to run as soon as the page loads. To do that we need to call the function in the ngOnInit()
like this:
ngOnInit(): void {
this.createChart();
}
Finally, we need to add the HTML selector of the bar-chart component to the app.component.html
file. Remove the initial Angular template code (if you created a new app) and add the following:
<app-bar-chart></app-bar-chart>
Creating a Line Chart with Angular Chart.js
A line chart connects several data points with a continuous line to graphically depict the historical value movement of an item. This is one of the simple charts which developers use in finance, and it often only shows the closing prices of an asset over time. Line charts developer also use them for any timescale; however, most often, for changes in daily prices in finance.
Adding a Line chart
Paste the same code for createChart() method on the line-chart.component.ts file below the ngOnInit() function. You just need to change the keyword for the type of chart from bar to line.
createChart(){
this.chart = new Chart("MyChart", {
type: 'line', //this denotes tha type of chart
data: {// values on X-Axis
labels: ['2022-05-10', '2022-05-11', '2022-05-12','2022-05-13', '2022-05-14', '2022-05-15', '2022-05-16','2022-05-17'],
datasets: [
{
label: "Sales",
data: ['467','576', '572', '79', '92','574','573','576'],
backgroundColor: 'green'
},
{
label: "Profit",
data: ['542', '542', '536', '327', '17','0.00','538', '541'],
backgroundColor: 'blue'
}
]
},
options: {
aspectRatio:2.5
}
});
}
Call the createChart() function in ngOnInit() and your line chart is ready.
ngOnInit(): void {
this.createChart();
}
Finally, we need to add the HTML selector of the line-chart component to the app.component.html file.
<app-line-chart></app-line-chart>
Final word on Angular Chart.js
And there you have it! You have just implemented basic Chart.js charts in Angular in less than 1 hour (I assume xD). From this point onward, it will be enough for you to follow documentation from Chart.js for additional options. Don’t forget to replace the dummy data with the real values you receive from the server! Thanks for reading, check out another cool article: Angular Load More Data on Button Click
Leave a Reply