It is finally here! New major Angular 15 version with many new features is here, as planned. This time, Angular team delivered some important bug fixes and tools to make our programming day job much simpler. There are some performance improvements as well, so let’s get started in listing only the biggest changes one by one:
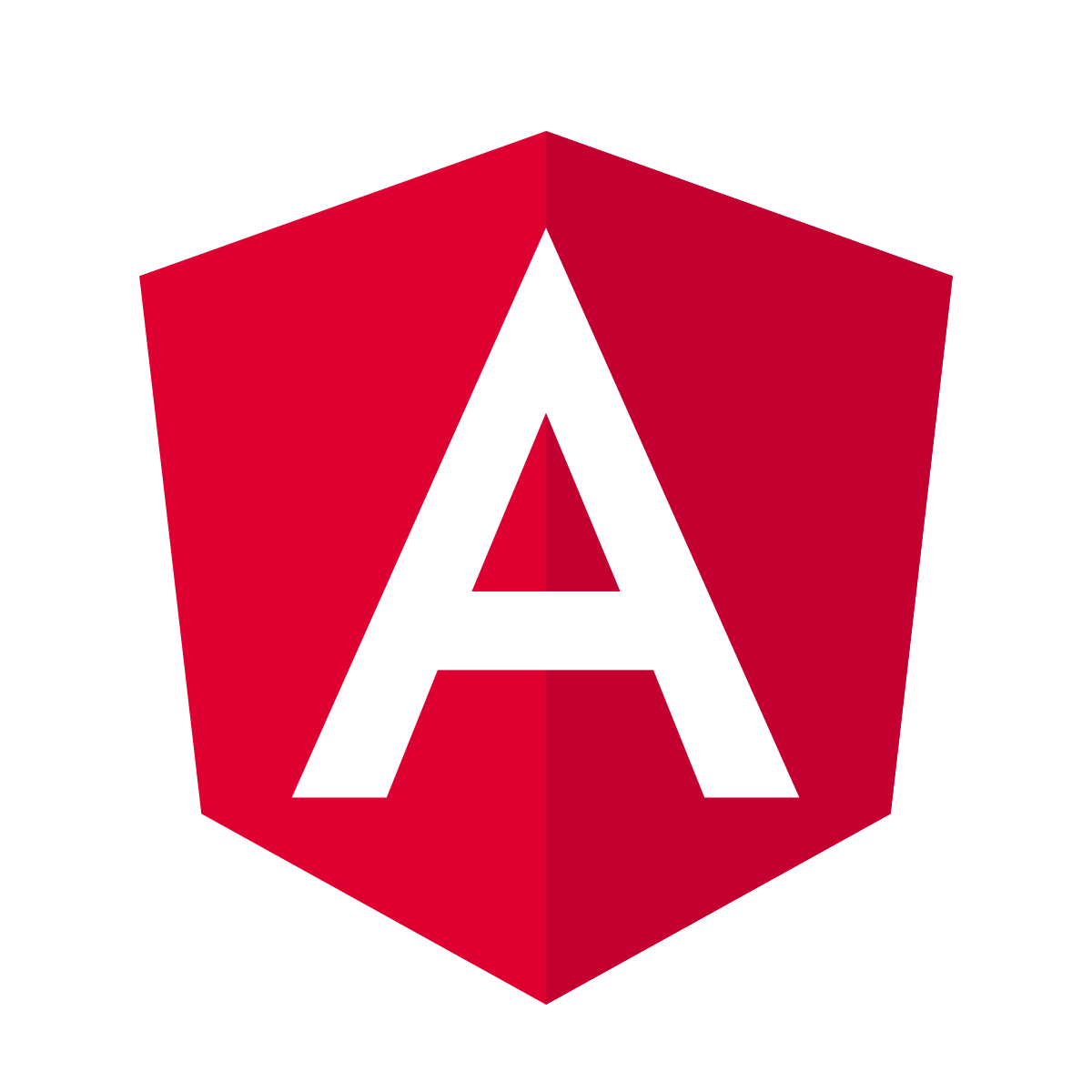
Standalone Components, Directives and Pipes
The previous release of Angular (version 14) brought Standalone APIs to developers, but this version 15 is bringing them to the public! What is meant my standalone APIs? That is a removed required need of NgModules
! Imagine creating a component or a pipe and not having to put it in the declarations array of NgModule. We have that now! To be able to do that, just mark the component, directive or pipe with standalone: true
and you don’t have to import it into any module. Let’s see an example:
@Component({
standalone: true,
selector: 'app-header',
template: `
<h1>Header</h1>`,
})
export class HeaderComponent{
}
Tree-shakable Router and HttpClient
New Router standalone APIs are here as well. Tree-shakable concept means that your dead code is automatically removed. So just by using this new API you will automatically gain performance benefits if you have dead code in your application. Don’t worry, dead code happens to great developers as well! Angular team found out this approach reduces 11% of router code!
Let me show you how to use this new API.
First, declare the root route:
export const appRoutes: Routes = [{
path: 'lazy',
loadChildren: () => import('./lazy/lazy.routes')
.then(routes => routes.lazyRoutes)
}];
Where lazy.routes
are:
import {Routes} from '@angular/router';
import {LazyComponent} from './lazy.component';
export const lazyRoutes: Routes = [{path: '', component: LazyComponent}];
Finally, provide appRoutes
in the bootstrapApplication
call:
bootstrapApplication(AppComponent, {
providers: [
provideRouter(appRoutes)
]
});
Stable Image directive
Image directive is coming out of developer preview and coming to public! As Land’s End claim, the improvement in LCP is 75% in a lighthouse test and that is a very good reason to consider using this in your projects. Just import NgOptimizedImage
in your module and use the directive like this:
<img ngSrc="angular_15.jpg" alt="Angular 15">
As you can see, the only change is src to ngSrc in the image tag. Also, make sure to specify the priority
attribute for LCP images. Here is an example:
<img ngSrc="angular_15.jpg" alt="Angular 15" priority>
Improved Stack traces
Angular partnered with Chrome DevTools to fix bad and ambiguous stack traces. Here is a stack trace you have probably seen tens of times:
ERROR Error: Uncaught (in promise): Error
Error
at app.component.ts:16:11
at Generator.next (<anonymous>)
at asyncGeneratorStep (asyncToGenerator.js:3:1)
at _next (asyncToGenerator.js:25:1)
at _ZoneDelegate.invoke (zone.js:372:26)
at Object.onInvoke (core.mjs:26378:33)
at _ZoneDelegate.invoke (zone.js:371:52)
at Zone.run (zone.js:134:43)
at zone.js:1275:36
at _ZoneDelegate.invokeTask (zone.js:406:31)
at resolvePromise (zone.js:1211:31)
at zone.js:1118:17
at zone.js:1134:33
Now, in the Angular 15 version in Chrome DevTools, the stack trace above is simplified as follows:
ERROR Error: Uncaught (in promise): Error
Error
at app.component.ts:16:11
at fetch (async)
at (anonymous) (app.component.ts:4)
at request (app.component.ts:4)
at (anonymous) (app.component.ts:15)
at submit (app.component.ts:14)
at AppComponent_click_3_listener (app.component.html:4)
End of major Angular 15 changes
That would be the list of biggest changes in my opinion. Too see all changes, read more on Angular Blog or view Angular 15 Release on GitHub. You can also have a look at my Angular 15 Crash Course with Angular Best Practices Ebook included for free!
Leave a Reply