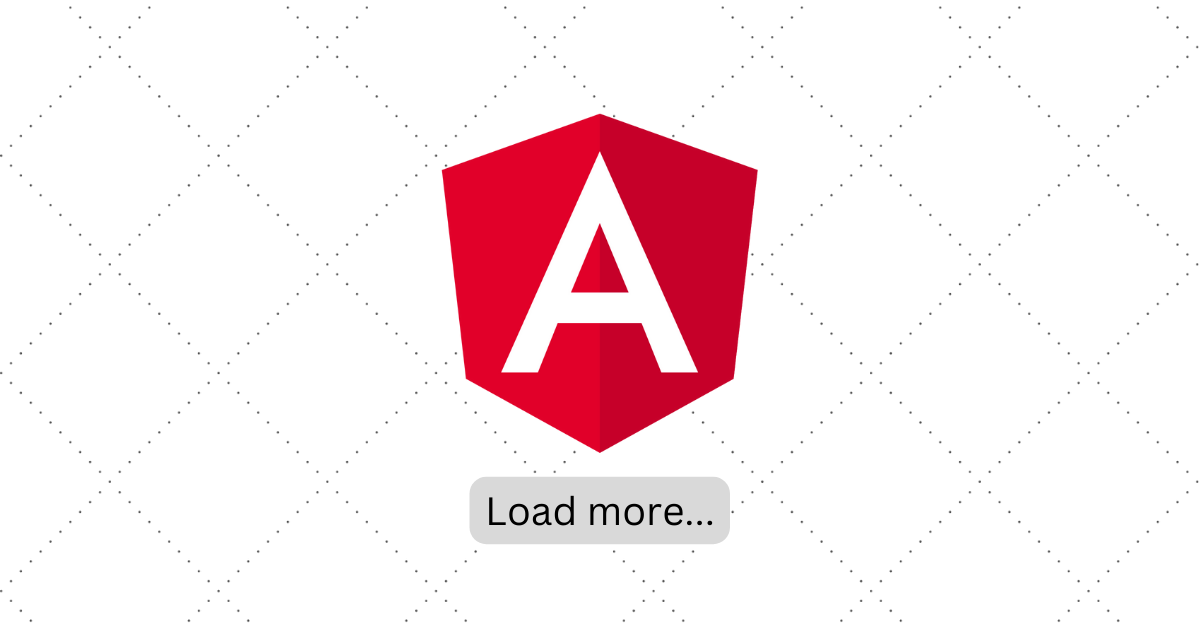
Hey there, here is an interesting one about Angular Load more button:
You have probably found yourself in a situation where you need to show only X number of records and hide the rest. Then, on a click of some kind of a Load more button, the next X number of records are shown until there are no more records to be shown. This article is going to go through several ideas how to solve this problem.
Approach 1: Two arrays
The first idea that comes to mind is to have two arrays: one that will contain all the elements, and one that will have all the elements.
Let’s say we want to display 10 items at a time. We would have:
public page = 0;
public allItems = [];
public shownItems = [];
public readonly numberOfRecordsOffset = 10;
After loading the data in the allItems array, we need to populate the first 10 items in shownItems array:
public loadMoreButtonClicked(): void {
let offset = this.page * this.numberOfRecordsOffset;
for (let i = offset; i < offset + this.numberOfRecordsOffset; i++) {
if (this.allItems[i]) {
this.shownItems.push(this.allItems[i]);
}
}
this.page += 1;
}
As you can see from above, we start to get the first 10 elements and every new function call increases page by 1, so the offset is respectively: 10, 20, 30, 40, etc. We can also graciously handle the visibility of Load more button by defining a flag that will keep Load more button visible while allItems array has more elements than shownItems array,
public isLoadMoreButtonVisible = this.allItems.length > this.shownItems.length;
Approach 2: Slice Pipe (recommended Angular Load more button approach)
I recently used this approach and it is the reason why I wrote this article in the first place. For this approach we will need only 1 array, 1 constant and 1 variable. I will use them to store the number of total records to be displayed. Moreover, we can also have a flag that will determine whether the Load more button should be visible or not.
public items = [];
public isLoadMoreVisible = false;
public readonly numberOfRecordsOffset = 10;
public totalNumberOfRecordsToShow = this.numberOfRecordsOffset;
The idea is to to populate items array with all data and use pipe to show the data in the template. Let’s see how the function to populate data should look like:
private populateData(data: CustomData): void {
this.items = data;
this.isLoadMoreVisible = this.items.length > this.numberOfRecordsOffset;
}
We assign isLoadMoreVisible‘s iniital value here to see whether we need this button at all.
Then, in the component’s template, we will make use of Angular slice pipe like this:
<item-card
*ngFor="let item of items | slice: 0: totalNumberOfRecordsToShow"
>
</item-card>
Finally, we need to create a loadMoreButtonClick() method to define what will happen on that button click including modifying totalNumberOfRecordsToShow and isLoadMoreVisible:
public loadMoreButtonClicked(): void {
this.totalNumberOfRecordsToShow += numberOfRecordsOffset;
this.isLoadMoreVisible = this.totalNumberOfRecordsToShow < this.items.length;
}
And that’s it! Each click on Load more button will check whether Load more button is visible. Moreover, it will change the total number of records that should be displayed in the UI. The value will respectively increase by 10. So, the slice will show elements from 0 to 10, then from 0 to 20, 0 to 30, etc.
Check out homepage for the newest articles!
Leave a Reply